更新购物车商品数量
用户点击商品数量加减按钮,向后端发送请求,更新购物车数据表
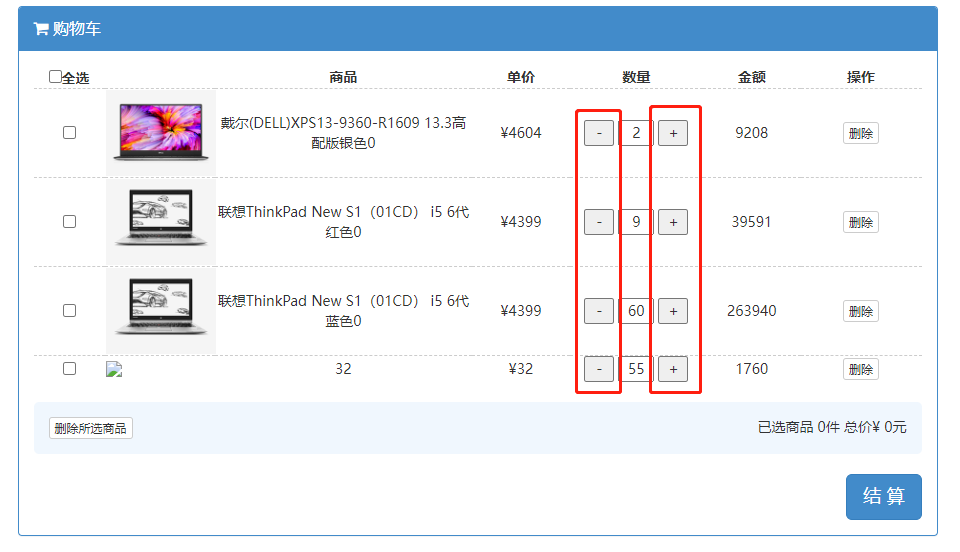
持久层
规划执行的SQL语句
本质是更新语句,更具cid跟新num字段值,在前面功能模块中已经实现
在执行更新操作之前,还需要判断当前购物车记录在数据库表中是否存在,本质是查询语句
1
| select * from t_cart where cid=?;
|
接口和抽象方法
1 2 3 4 5 6
|
Cart findByCid(Integer cid);
|
SQL映射文件配置
1 2 3
| <select id="findByCid" resultMap="cartPojoMap"> select * from t_cart where cid=#{cid}; </select>
|
单元测试
1 2 3 4 5
| @Test public void findByCid(){ Cart cart = cartMapper.findByCid(1); System.out.println(cart); }
|
业务层
规划异常
1.数据更新过程中会发生异常,此异常类在此前的功能模块中已经定义
2.查询数据与登录用户不匹配引发的异常,此异常在此前功能模块中也已经定义
3.查询的购物车记录在数据库中不存在,创建对应的异常类CartNotFoundException
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.bang.store.service.ex;
public class CartNotFoundException extends ServiceException{ public CartNotFoundException() { super(); }
public CartNotFoundException(String message) { super(message); }
public CartNotFoundException(String message, Throwable cause) { super(message, cause); }
public CartNotFoundException(Throwable cause) { super(cause); }
protected CartNotFoundException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) { super(message, cause, enableSuppression, writableStackTrace); } }
|
接口和抽象方法
1 2 3 4 5 6 7 8 9
|
Integer alterNum(Integer cid,Integer uid,String username,Integer changeNum);
|
抽象方法实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| @Override public Integer alterNum(Integer cid, Integer uid, String username, Integer changeNum) { Cart result = cartMapper.findByCid(cid); if(result==null){ throw new CartNotFoundException("数据库该数据不存在"); } if(!result.getUid().equals(uid)){ throw new AccessDeniedException("非法数据访问"); } Integer rows = cartMapper.updateNumByCid(cid, result.getNum() + changeNum, username, new Date()); if(rows!=1){ throw new UpdateException("数据更新过程发生未知错误"); } return result.getNum()+changeNum; }
|
单元测试
1 2 3 4 5
| @Test public void alertNum(){ Integer num = cartService.alterNum(1, 1, "admin", -20); System.out.println(num); }
|
控制层
处理异常
在控制层基类BaseController
中添加购物车数据不存在异常CartNotFoundException
1 2 3 4
| else if (e instanceof CartNotFoundException) { result.setState(9000); result.setMessage("购物车数据不存在"); }
|
设计请求
1 2 3 4
| request url: /cart/alter_num/{cid}/{opcode} //restful数据访问,cid代表购物车数据id,opcode:1代表增加商品数量,0代表较小商品数量 request method: GET request params: Integer cid,Integr opcode,HttpSession session response data: new JsonResult<Integer>
|
处理请求
1 2 3 4 5 6 7 8 9 10 11 12
| @RequestMapping("/alter_num/{cid}/{opcode}") public JsonResult<Integer> alterNum(@PathVariable Integer cid,@PathVariable Integer opcode,HttpSession session){ Integer uid = getUidFromSession(session); String username = getUsernameFromSession(session); Integer num = -1; if(opcode==0){ num = cartService.alterNum(cid,uid,username,-1); } else if (opcode==1) { num = cartService.alterNum(cid,uid,username,1); } return new JsonResult<>(OK,"商品数量改变成功",num); }
|
前端页面
在cart.html
页面点击增加或者减少按钮,改变购物车中对应商品的数量
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68
| function showCartList(){ $.ajax({ url: "/cart/" ,type: "GET" ,success: function (data){ if(data.state == 200){ alert("购物车数据获取成功"); $("#cart-list").empty(); cartList = data.data; for(let i=0;i<cartList.length;i++){ htmlContent = "<tr>\n" + "<td>\n" + "<input name=\"cids\" value='#{cid}' type=\"checkbox\" class=\"ckitem\" />\n" + "</td>\n" + "<td><img src=\"..#{image}collect.png\" class=\"img-responsive\" /></td>\n" + "<td>#{title}</td>\n" + "<td>¥<span id=\"goodsPrice#{cid}\">#{price}</span></td>\n" + "<td>\n" + '<input id=\"price-#{cid}\" type=\"button\" value=\"-\" class=\"num-btn\" onclick="chageNum(#{cid},0)"/>\n' + "<input id=\"goodsCount#{cid}\" type=\"text\" size=\"2\" readonly=\"readonly\" class=\"num-text\" value=\"#{num}\">\n" + "<input id=\"price+#{cid}\" class=\"num-btn\" type=\"button\" value=\"+\" onclick=\"chageNum(#{cid},1)\"/>\n" + "</td>\n" + "<td><span id=\"goodsCast#{cid}\">#{totalPrice}</span></td>\n" + "<td>\n" + "<input type=\"button\" class=\"cart-del btn btn-default btn-xs\" value=\"删除\" />\n" + "</td>\n" + "</tr>"; htmlContent = htmlContent.replaceAll("#{cid}",cartList[i].cid); htmlContent = htmlContent.replace("#{image}",cartList[i].productImage); htmlContent = htmlContent.replace("#{title}",cartList[i].productTitle+(cartList[i].cartPrice-cartList[i].productPrice)); htmlContent = htmlContent.replace("#{num}",cartList[i].cartNum); htmlContent = htmlContent.replace("#{price}",cartList[i].cartPrice); htmlContent = htmlContent.replace("#{totalPrice}",cartList[i].cartNum*cartList[i].cartPrice); $("#cart-list").append(htmlContent); }
}else{ alert("购物车获取数据失败 "+data.message); } } ,error:function (xmh){ alert("购物车获取数据发生未知异常"+xmh.status); } }); }
function chageNum(cid,opcode){ $.ajax({ url: "/cart/alter_num/"+cid+"/"+opcode ,type: "GET" ,dataType: "JSON" ,success: function (data){ if(data.state == 200){ $("#goodsCount"+cid).val(data.data); let price = $("#goodsPrice"+cid).html(); let totalPrice = price*data.data; $("#goodsCast"+cid).html(totalPrice); alert("购物车商品数量修改成功"); }else{ alert("购物车商品数量修改失败 "+data.message); } } ,error:function (xmh){ alert("购物车商品数量修改发生未知异常"+xmh.status); } }); }
|