收货地址删除
用户点击删除按钮,向后端发送请求,删除用户该条地址记录,同时前端页面该地址删除
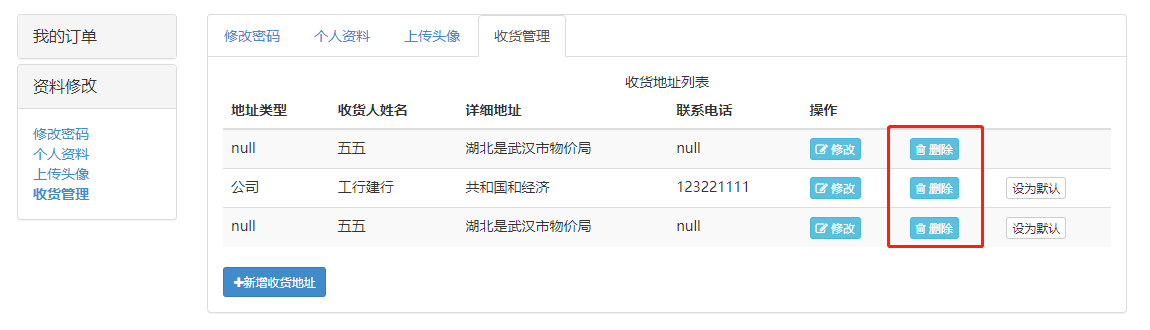
持久层
规划执行的SQL语句
1.删除该地址之前需要判断该数据在数据库中是否存在,此功能在设置默认地址模块已经实现
2.判断该条地址是否与当前登录用户相匹配,此功能在设置默认地址模块也已经实现
3.删除对应的地址数据
1
| delete from t_address where aid=?;
|
4.如果用户删除的是默认收货地址,则将剩下的地址中的某一条设置为新的默认地址,规则可以自定义:将最新修改时间的收货地址设置为默认的收货地址
1
| select * from t_address where uid=? order by modified_time DESC limit 0,1;
|
5.如果用户本身只有一条收货地址,删除之后,不用进行后续操作;查询用户地址数目语句在前面新增地址功能模块已经实现过了
接口和抽象方法
在AddressMapper
类中定义对应抽象方法
1 2 3 4 5 6 7 8 9 10 11 12 13
|
Integer deleteByAid(Integer aid);
Address findLastModified(Integer uid);
|
SQL映射文件配置
在AddressMapper.xml
中编写对应的SQL映射语句
1 2 3 4 5 6 7
| <delete id="deleteByAid"> delete from t_address where aid=#{aid}; </delete>
<select id="findLastModified" resultMap="addressPojoMap"> select * from t_address where uid=#{uid} order by modified_time DESC limit 0,1; </select>
|
单元测试
1 2 3 4 5 6 7 8 9 10 11 12
| @Test public void deleteByAid(){ Integer aid = 9; Integer rows = addressMapper.deleteByAid(aid); System.out.println(rows); } @Test public void findLastModified(){ Address address = addressMapper.findLastModified(3); System.out.println(address); System.out.println(address.getModifiedTime()); }
|
业务层
规划异常
当前数据不存在异常;地址数据与用户不匹配异常在之前功能模块中已经定义过了
删除过程中可能发生未知错误引发异常,定义对应的异常类DeleteException
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.bang.store.service.ex;
public class DeleteException extends ServiceException{ public DeleteException() { super(); }
public DeleteException(String message) { super(message); }
public DeleteException(String message, Throwable cause) { super(message, cause); }
public DeleteException(Throwable cause) { super(cause); }
protected DeleteException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) { super(message, cause, enableSuppression, writableStackTrace); } }
|
接口和抽象方法
在IAddressService
接口中编写对应的抽象方法
1 2 3 4 5 6 7
|
void delete(Integer aid,Integer uid,String username);
|
抽象方法实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| @Override public void delete(Integer aid, Integer uid, String username) { Address address = addressMapper.findByAid(aid); if(address==null){ throw new AddressNotFoundException("地址数据不存在"); } if(!address.getUid().equals(uid)){ throw new AccessDeniedException("非法数据访问"); } Integer rows = addressMapper.deleteByAid(aid); if(rows!=1){ throw new DeleteException("删除过程发生未知错误"); }
if(address.getIsDefault()==0) return; Integer count = addressMapper.countByUid(uid); if(count>0){ Address lastModifiedAddress = addressMapper.findLastModified(uid); rows = addressMapper.updateDefaultByAid(lastModifiedAddress.getAid(), username, new Date()); if(rows!=1){ throw new UpdateException("数据更新发生未知异常"); } } }
|
单元测试
1 2 3 4 5 6 7
| @Test public void delete(){ Integer aid = 7; Integer uid=3; String username="管理员二号"; addressService.delete(aid,uid,username); }
|
控制层
异常处理
业务层新增DeleteException
,在控制基类BaseController
中添加对应的异常处理逻辑
1 2 3 4
| else if (e instanceof DeleteException){ result.setState(7003); result.setMessage("数据删除过程发生未知错误"); }
|
设计请求
1 2 3 4
| request url: /address/delete/{aid} #restful风格数据请求 request method: GET request params: Integer aid,HttpSession session response data: new JsonResult<Void>()
|
请求处理
1 2 3 4 5 6 7
| @RequestMapping("/delete/{aid}") public JsonResult<Void> delete(@PathVariable("aid") Integer aid,HttpSession session){ Integer uid = getUidFromSession(session); String username = getUsernameFromSession(session); addressService.delete(aid,uid,username); return new JsonResult<>(OK,"地址数据删除成功"); }
|
前端页面
在address.html
编写对应的页面逻辑
在删除按钮组件添加onclick
属性,绑定对应删除逻辑函数
组件添加onclick
属性,绑定事件处理函数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| function showAddress() { $.ajax({ url: "/address/get_by_uid" ,type: "GET" ,data: null ,dataType: "JSON" ,success: function (data){ if(data.state == 200){ $("#address-list").empty(); let addressList = data.data; for(let i=0;i<addressList.length;i++){ let trContent = "<tr>\n" + "\t\t\t\t\t\t\t\t\t<td>"+addressList[i].tag+"</td>\n" + "\t\t\t\t\t\t\t\t\t<td>"+addressList[i].name+"</td>\n" + "\t\t\t\t\t\t\t\t\t<td>"+addressList[i].address+"</td>\n" + "\t\t\t\t\t\t\t\t\t<td>"+addressList[i].phone+"</td>\n" + "\t\t\t\t\t\t\t\t\t<td><a class=\"btn btn-xs btn-info\"><span class=\"fa fa-edit\"></span> 修改</a></td>\n" + "\t\t\t\t\t\t\t\t\t<td><a onclick='del(#{aid})' class=\"btn btn-xs add-del btn-info\"><span class=\"fa fa-trash-o\"></span> 删除</a></td>\n" + "\t\t\t\t\t\t\t\t\t<td><a onclick='setDefault(#{aid})' class=\"btn btn-xs add-def btn-default\">设为默认</a></td>\n" + "\t\t\t\t\t\t\t\t</tr>"; trContent = trContent.replace("#{aid}",addressList[i].aid); $("#address-list").append(trContent); } $(".add-def:eq(0)").hide(); }else{ alert("用户地址获取失败 "+data.message); } } ,error:function (xmh){ alert("用户地址获取过程中发生未知错误"+xmh.status); } });
|
编写删除按钮事件处理函数del
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| function del(aid) { $.ajax({ url: "/address/delete/"+aid ,type: "GET" ,success: function (data){ if(data.state == 200){ alert("删除地址成功"); showAddress() }else{ alert("删除地址失败 "+data.message); } } ,error:function (xmh){ alert("删除地址发生未知错误"+xmh.status); } }); }
|